-
Notifications
You must be signed in to change notification settings - Fork 4
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
- Loading branch information
Showing
28 changed files
with
398 additions
and
280 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -1,2 +1,3 @@ | ||
node_modules | ||
package-lock.json | ||
dist | ||
package-lock.json |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,6 @@ | ||
/node_modules | ||
/src | ||
/tests | ||
|
||
tsconfig.json | ||
tsup.config.ts |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -1,72 +1,135 @@ | ||
# **About** | ||
**Greetify** is futuristic welcome card canvas library | ||
<img src="https://ik.imagekit.io/unburn/greetify.svg"/> | ||
|
||
# **Installation** | ||
<p align="center">Futuristic welcome card canvas library</p> | ||
|
||
<p align="center"> | ||
<a href="https://github.com/unburn/greetify"><b>Github</b></a> • | ||
<a href="https://discord.gg/66uGX7t4ww"><b>Support</b></a> | ||
</p> | ||
|
||
<div align="center"> | ||
|
||
[](https://www.npmjs.com/package/greetify) | ||
[](https://www.npmjs.com/package/greetify) | ||
[](https://github.com/unburn/greetify/blob/main/LICENCE) | ||
[](https://github.com/unburn/greetify) | ||
|
||
</div> | ||
|
||
<div align="center"> | ||
<a href="https://github.com/sponsors/flameface"><img src="https://ik.imagekit.io/unburn/support-greetify.svg?updatedAt=1710761418683"/></a> | ||
</div> | ||
|
||
# Installation | ||
``` | ||
npm i greetify | ||
npm install greetify | ||
``` | ||
|
||
# **Example Usage** | ||
This example code will create a welcome card and save it as a png. | ||
```js | ||
(async () => { | ||
// Importing modules | ||
const { welcomeCard } = require("greetify"); | ||
const fs = require("fs") | ||
|
||
// Card details here | ||
const card = new welcomeCard() | ||
.setName("FlameFace") | ||
.setAvatar("https://s6.imgcdn.dev/ZFQlq.png") | ||
.setMessage("YOU ARE 688 MEMBER") | ||
.setBackground("https://s6.imgcdn.dev/ZqH2S.png") | ||
.setColor("00FF38") // without # | ||
.setTitle("Welcome") | ||
|
||
// Building process | ||
const output = await card.build(); | ||
|
||
// Save as image | ||
fs.writeFileSync("card.png", output); | ||
console.log("Done"); | ||
})() | ||
# Usage | ||
|
||
## Using File System (FS) | ||
```javascript | ||
import { Panorama } from "greetify"; | ||
import fs from "fs"; | ||
|
||
// OR | ||
|
||
const { Panorama } = require("greetify"); | ||
const fs = require('fs') | ||
|
||
Panorama({ | ||
avatar: "https://cdn.discordapp.com/avatars/786504767358238720/f65e8322c0c290e7fc1d9ad20322256b.webp", | ||
name: "FLAMEFACE", | ||
type: "WELCOME", | ||
}).then(x => { | ||
fs.writeFileSync("greetify.png", x) | ||
}) | ||
``` | ||
|
||
This example is of **Discord Bot** using discord.js | ||
```js | ||
// Importing modules | ||
const { welcomeCard } = require("greetify"); | ||
const { AttachmentBuilder } = require("discord.js") | ||
|
||
// Make sure to define client | ||
client.on('guildMemberAdd', async (member) => { | ||
// Card details here | ||
const card = new welcomeCard() | ||
.setName("FlameFace") | ||
.setAvatar("https://s6.imgcdn.dev/ZFQlq.png") | ||
.setMessage("YOU ARE 688 MEMBER") | ||
.setBackground("https://s6.imgcdn.dev/ZqH2S.png") | ||
.setColor("00FF38") // without # | ||
.setTitle("Welcome") | ||
|
||
// Building process | ||
const output = await card.build() | ||
|
||
// Fetch channel from members guild using ID | ||
const channel = member.guild.channels.cache.get("0000000000000000000"); | ||
|
||
// Sends card to the "channel" | ||
await channel.send({ | ||
## In Discord Bot | ||
```javascript | ||
// Assuming you defined client | ||
const { Minimal } = require("greetify"); | ||
|
||
client.on("guildMemberAdd", async member => { | ||
const message = `YOU ARE ${member.guild.memberCount}TH MEMBER` | ||
|
||
const card = await Minimal({ | ||
name: member.user.username, | ||
avatar: member.user.displayAvatarURL({ | ||
size: 4096 // For High Res Avatar | ||
}), | ||
type: "WELCOME", | ||
message: message | ||
}) | ||
|
||
const channel = member.guild.channels.cache.get("1201155869610627212"); | ||
|
||
return channel.send({ | ||
files: [{ | ||
attachment: output, | ||
name: `${member.id}.png` | ||
attachment: card | ||
}] | ||
}) | ||
}) | ||
``` | ||
|
||
### **Output** | ||
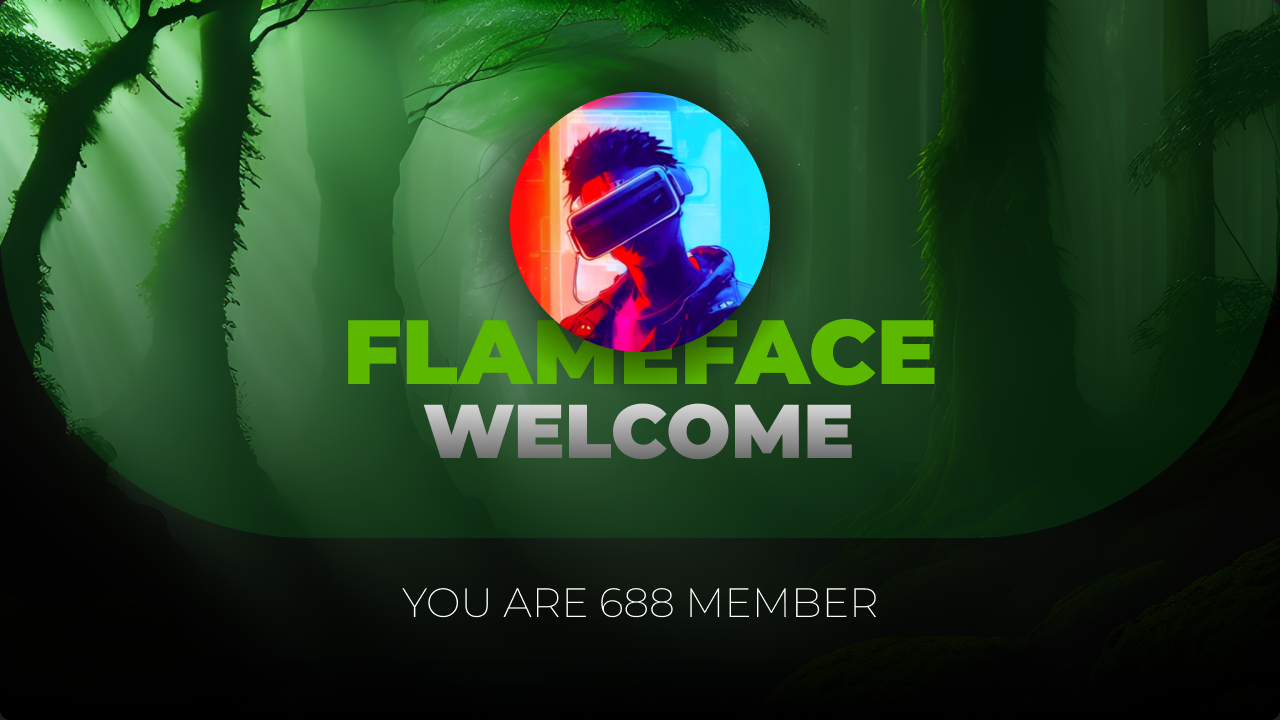 | ||
# Themes | ||
## Minimal | ||
 | ||
|
||
```javascript | ||
const { Minimal } = require("greetify"); | ||
const fs = require('fs') | ||
|
||
Minimal({ | ||
avatar: "https://cdn.discordapp.com/avatars/786504767358238720/f65e8322c0c290e7fc1d9ad20322256b.webp", | ||
name: "FLAMEFACE", | ||
type: "WELCOME" | ||
}).then(x => { | ||
fs.writeFileSync("greetify.png", x) | ||
}) | ||
``` | ||
|
||
### Minimal Options | ||
| Parameters | Types | Default | | ||
| --------------- | ------- | -------------------------------------------------- | | ||
| avatar* | string | none | | ||
| backgroundImage | string | https://ik.imagekit.io/unburn/greetify-default.png | | ||
| circleBorder | boolean | false | | ||
| message* | string | none | | ||
| messageColor | string | #FFFFFF | | ||
| name* | string | none | | ||
| nameColor | string | #00FF9E | | ||
| type | string | WELCOME | | ||
| typeColor | string | #FFFFFF | | ||
|
||
|
||
## Panorama | ||
 | ||
|
||
```javascript | ||
const { Panorama } = require("greetify"); | ||
const fs = require('fs') | ||
|
||
Panorama({ | ||
avatar: "https://cdn.discordapp.com/avatars/786504767358238720/f65e8322c0c290e7fc1d9ad20322256b.webp", | ||
name: "FLAMEFACE", | ||
type: "WELCOME" | ||
}).then(x => { | ||
fs.writeFileSync("greetify.png", x) | ||
}) | ||
``` | ||
|
||
### Panorama Options | ||
| Parameters | Types | Default | | ||
| --------------- | ------- | -------------------------------------------------- | | ||
| avatar* | string | none | | ||
| backgroundImage | string | https://ik.imagekit.io/unburn/greetify-default.png | | ||
| circleBorder | boolean | false | | ||
| name* | string | none | | ||
| nameColor | string | #00FF9E | | ||
| type | string | WELCOME | | ||
| typeColor | string | #FFFFFF | | ||
|
||
# **Help** | ||
If you need help or want some features to be added, join our official **[burnxpofficial](https://discord.gg/qDysF95NWh)** community. | ||
# Licence | ||
[GPL](https://github.com/unburn/greetify/blob/main/LICENCE) |
This file was deleted.
Oops, something went wrong.
This file was deleted.
Oops, something went wrong.
Binary file not shown.
Binary file not shown.
This file was deleted.
Oops, something went wrong.
Binary file not shown.
Oops, something went wrong.