diff --git a/ImageReport.docx b/ImageReport.docx new file mode 100644 index 00000000..c815bf59 Binary files /dev/null and b/ImageReport.docx differ diff --git a/Project_Presentation.pptx b/Project_Presentation.pptx new file mode 100644 index 00000000..687e97a3 Binary files /dev/null and b/Project_Presentation.pptx differ diff --git a/README.md b/README.md index 65596e0e..2848ded5 100644 --- a/README.md +++ b/README.md @@ -1,86 +1,50 @@ -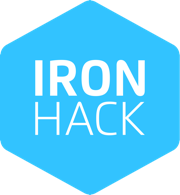 - -# Project I | Deep Learning: Image Classification with CNN - -## Task Description - -Students will build a Convolutional Neural Network (CNN) model to classify images from a given dataset into predefined categories/classes. - -## Datasets (pick one!) - -1. The dataset for this task is the CIFAR-10 dataset, which consists of 60,000 32x32 color images in 10 classes, with 6,000 images per class. You can download the dataset from [here](https://www.cs.toronto.edu/~kriz/cifar.html). -2. The second dataset contains about 28,000 medium quality animal images belonging to 10 categories: dog, cat, horse, spyder, butterfly, chicken, sheep, cow, squirrel, elephant. The link is [here](https://www.kaggle.com/datasets/alessiocorrado99/animals10/data). - -## Assessment Components - -1. **Data Preprocessing** - - Data loading and preprocessing (e.g., normalization, resizing, augmentation). - - Create visualizations of some images, and labels. - -2. **Model Architecture** - - Design a CNN architecture suitable for image classification. - - Include convolutional layers, pooling layers, and fully connected layers. - -3. **Model Training** - - Train the CNN model using appropriate optimization techniques (e.g., stochastic gradient descent, Adam). - - Utilize techniques such as early stopping to prevent overfitting. - -4. **Model Evaluation** - - Evaluate the trained model on a separate validation set. - - Compute and report metrics such as accuracy, precision, recall, and F1-score. - - Visualize the confusion matrix to understand model performance across different classes. - -5. **Transfer Learning** - - Evaluate the accuracy of your model on a pre-trained models like ImagNet, VGG16, Inception... (pick one an justify your choice) - - You may find this [link](https://www.tensorflow.org/tutorials/images/transfer_learning_with_hub) helpful. - - [This](https://pytorch.org/tutorials/beginner/transfer_learning_tutorial.html) is the Pytorch version. - - Perform transfer learning with your chosen pre-trained models i.e., you will probably try a few and choose the best one. - -5. **Code Quality** - - Well-structured and commented code. - - Proper documentation of functions and processes. - - Efficient use of libraries and resources. - -6. **Report** - - Write a concise report detailing the approach taken, including: - - Description of the chosen CNN architecture. - - Explanation of preprocessing steps. - - Details of the training process (e.g., learning rate, batch size, number of epochs). - - Results and analysis of models performance. - - What is your best model. Why? - - Insights gained from the experimentation process. - - Include visualizations and diagrams where necessary. - - 7. **Model deployment** - - Pick the best model - - Build an app using Flask - Can you host somewhere other than your laptop? **+5 Bonus points if you use [Tensorflow Serving](https://www.tensorflow.org/tfx/guide/serving)** - - User should be able to upload one or multiples images get predictions including probabilities for each prediction - - -## Evaluation Criteria - -- Accuracy of the trained models on the validation set. **30 points** -- Clarity and completeness of the report. **20 points** -- Quality of code implementation. **5 points** -- Proper handling of data preprocessing and models training. **30 points** -- Demonstration of understanding key concepts of deep learning. **5 points** -- Model deployment. **10 points** - - **Passing Score is 70 points**. - -## Submission Details - -- Deadline for submission: end of the week or as communicated by your teaching team. -- Submit the following: - 1. Python code files (`*.py`, `ipynb`) containing the model implementation and training process. - 2. A data folder with 5-10 images to test the deployed model/app if hosted somewhere else other than your laptop (strongly recommended! Not a must have) - 2. A PDF report documenting the approach, results, and analysis. - 3. Any additional files necessary for reproducing the results (e.g., requirements.txt, README.md). - 4. PPT presentation - -## Additional Notes - -- Students are encourage to experiment with different architectures, hyper-parameters, and optimization techniques. -- Provide guidance and resources for troubleshooting common issues during model training and evaluation. -- Students will discuss their approaches and findings in class during assessment evaluation sessions. - +# Image Classifier + +This is a simple image classification application using Flask for the backend and a Tailwind CSS-based UI. It allows users to upload an image and get predictions from a pre-trained TensorFlow model. + +--- + +## Features +- Upload images via a user-friendly web interface. +- Classify images using a pre-trained TensorFlow model. +- Display prediction probabilities for each class. + +--- + +## Requirements +- Python 3.8, 3.9, or 3.10 +- Pip (Python package installer) + +--- + +## Installation and Setup Instructions + +1. **Install Python Dependencies** + Run the following command to install all required Python libraries: + - pip install flask tensorflow pillow + +--- + +2. **Download the Pre-trained Model** + Ensure your TensorFlow model file (`Final.keras`) is located at the following path: + - model\Final.keras + +--- + +3. **Open the Application** + Open your browser and navigate to: + - http://localhost:5000 + +--- + +## Usage + +1. Select an image file by clicking the "Choose File" button in the UI. +2. Click "Upload and Classify" to send the image to the server. +3. View the predicted class and probabilities for all classes in the UI. + +--- + +### Error: TensorFlow Compatibility +Ensure you are using Python 3.8, 3.9, or 3.10. TensorFlow may not work with other Python versions. + diff --git a/client.py b/client.py new file mode 100644 index 00000000..2cf16724 --- /dev/null +++ b/client.py @@ -0,0 +1,26 @@ +# import requests + +# # Ruta de la imagen a enviar +# image_path = "C:/Users/matel/Desktop/a1.png" + +# # URL del servidor Flask +# url = "http://localhost:5000/predict" + +# try: +# # Abrir la imagen y enviar la solicitud +# with open(image_path, "rb") as file: +# files = {"file": file} +# response = requests.post(url, files=files) + +# # Verificar el estado de la respuesta +# if response.status_code == 200: +# result = response.json() +# print("Predicted Class:", result["predicted_class"]) +# print("Probabilities:") +# for class_name, prob in result["probabilities"].items(): +# print(f" {class_name}: {prob:.4f}") +# else: +# print(f"Error: {response.status_code}") +# print(response.json()) +# except Exception as e: +# print(f"Error while sending the request: {str(e)}") diff --git a/index.html b/index.html new file mode 100644 index 00000000..41718878 --- /dev/null +++ b/index.html @@ -0,0 +1,91 @@ + + +
+ + +