diff --git a/docs/build/build-on-layer-1/smart-contracts/building-cross-chain-contracts/xcm/integration/_category_.json b/docs/about/integrate-with-astar/_category_.json
similarity index 100%
rename from docs/build/build-on-layer-1/smart-contracts/building-cross-chain-contracts/xcm/integration/_category_.json
rename to docs/about/integrate-with-astar/_category_.json
diff --git a/docs/build/build-on-layer-1/smart-contracts/building-cross-chain-contracts/xcm/integration/add-to-portal.md b/docs/about/integrate-with-astar/add-to-portal.md
similarity index 100%
rename from docs/build/build-on-layer-1/smart-contracts/building-cross-chain-contracts/xcm/integration/add-to-portal.md
rename to docs/about/integrate-with-astar/add-to-portal.md
diff --git a/docs/build/build-on-layer-1/smart-contracts/building-cross-chain-contracts/xcm/integration/asset-registration.md b/docs/about/integrate-with-astar/asset-registration.md
similarity index 100%
rename from docs/build/build-on-layer-1/smart-contracts/building-cross-chain-contracts/xcm/integration/asset-registration.md
rename to docs/about/integrate-with-astar/asset-registration.md
diff --git a/docs/build/build-on-layer-1/smart-contracts/building-cross-chain-contracts/xcm/integration/hrmp.md b/docs/about/integrate-with-astar/hrmp.md
similarity index 100%
rename from docs/build/build-on-layer-1/smart-contracts/building-cross-chain-contracts/xcm/integration/hrmp.md
rename to docs/about/integrate-with-astar/hrmp.md
diff --git a/docs/build/build-on-layer-1/smart-contracts/building-cross-chain-contracts/xcm/integration/multilocation.md b/docs/about/integrate-with-astar/multilocation.md
similarity index 100%
rename from docs/build/build-on-layer-1/smart-contracts/building-cross-chain-contracts/xcm/integration/multilocation.md
rename to docs/about/integrate-with-astar/multilocation.md
diff --git a/docs/build/build-on-layer-1/smart-contracts/building-cross-chain-contracts/xcm/integration/tools.md b/docs/about/integrate-with-astar/tools.md
similarity index 100%
rename from docs/build/build-on-layer-1/smart-contracts/building-cross-chain-contracts/xcm/integration/tools.md
rename to docs/about/integrate-with-astar/tools.md
diff --git a/docs/build/build-on-layer-1/integrations/account-abstraction/_category_.json b/docs/build/build-on-layer-1/integrations/account-abstraction/_category_.json
new file mode 100644
index 0000000..ea891e3
--- /dev/null
+++ b/docs/build/build-on-layer-1/integrations/account-abstraction/_category_.json
@@ -0,0 +1,4 @@
+{
+ "label": "Account Abstraction",
+ "position": 1
+}
\ No newline at end of file
diff --git a/docs/build/build-on-layer-1/integrations/account-abstraction/banana/_category_.json b/docs/build/build-on-layer-1/integrations/account-abstraction/banana/_category_.json
new file mode 100644
index 0000000..2cb26bf
--- /dev/null
+++ b/docs/build/build-on-layer-1/integrations/account-abstraction/banana/_category_.json
@@ -0,0 +1,4 @@
+{
+ "label": "Banana Wallet SDK",
+ "position": 1
+}
\ No newline at end of file
diff --git a/docs/build/build-on-layer-1/integrations/account-abstraction/banana/index.md b/docs/build/build-on-layer-1/integrations/account-abstraction/banana/index.md
new file mode 100644
index 0000000..125a039
--- /dev/null
+++ b/docs/build/build-on-layer-1/integrations/account-abstraction/banana/index.md
@@ -0,0 +1,341 @@
+---
+sidebar_position: 3
+---
+# Banana SDK
+
+## Introduction
+In this tutorial we will show how you can integrate Banana Wallet to your JavaScript or TypeScript-based frontend. We will demonstrate how to create a new wallet or connect an existing Banana Wallet on any dApp on Astar Network.
+
+
+## Prerequisites
+
+ - Basic JavaScript/Typescript knowledge.
+ - Enthusiasm to build an amazing dApp on Astar.
+
+## Getting started
+
+### Step 1: Create a new repo with create-react-app
+Create a new react project with react with name `banana-sdk-demo` and now let's move into to the it.
+```
+npx create-react-app banana-sdk-demo
+cd banana-sdk-demo
+```
+
+### Step 2: Installing banana sdk package
+
+Install @rize-labs/banana-wallet-sdk package with
+
+```
+npm install @rize-labs/banana-wallet-sdk
+or
+yarn add @rize-labs/banana-wallet-sdk
+```
+
+### Step 3: Smart Contract deployment
+
+For this demo we will be using a very basic smart contract with only two functionalities:
+
+- Make a transaction to the blockchain by making a state variable change its value.
+- Fetch value of state variable.
+
+Code for smart contract
+
+```
+pragma solidity ^0.8.12;
+
+contract Sample {
+
+ uint public stakedAmount = 0;
+
+ function stake() external payable {
+ stakedAmount = stakedAmount + msg.value;
+ }
+
+ function returnStake() external {
+ payable(0x48701dF467Ba0efC8D8f34B2686Dc3b0A0b1cab5).transfer(stakedAmount);
+ }
+}
+```
+
+You can deploy the contract on Shibuya Testnet using [remix](https://remix.ethereum.org/) or something of your own choice.
+
+For this demo we had already deployed it here: `0xCC497f137C3A5036C043EBd62c36F1b8C8A636C0`
+
+### Step 4: Building the front end
+
+We will have a simple front end with some buttons to interact with the blockchain. Although Banana SDK provides you with a smart contract wallet you don't need worry about its deployment. Everything is handled by us in the SDK so you can concentrate on building your dApp.
+
+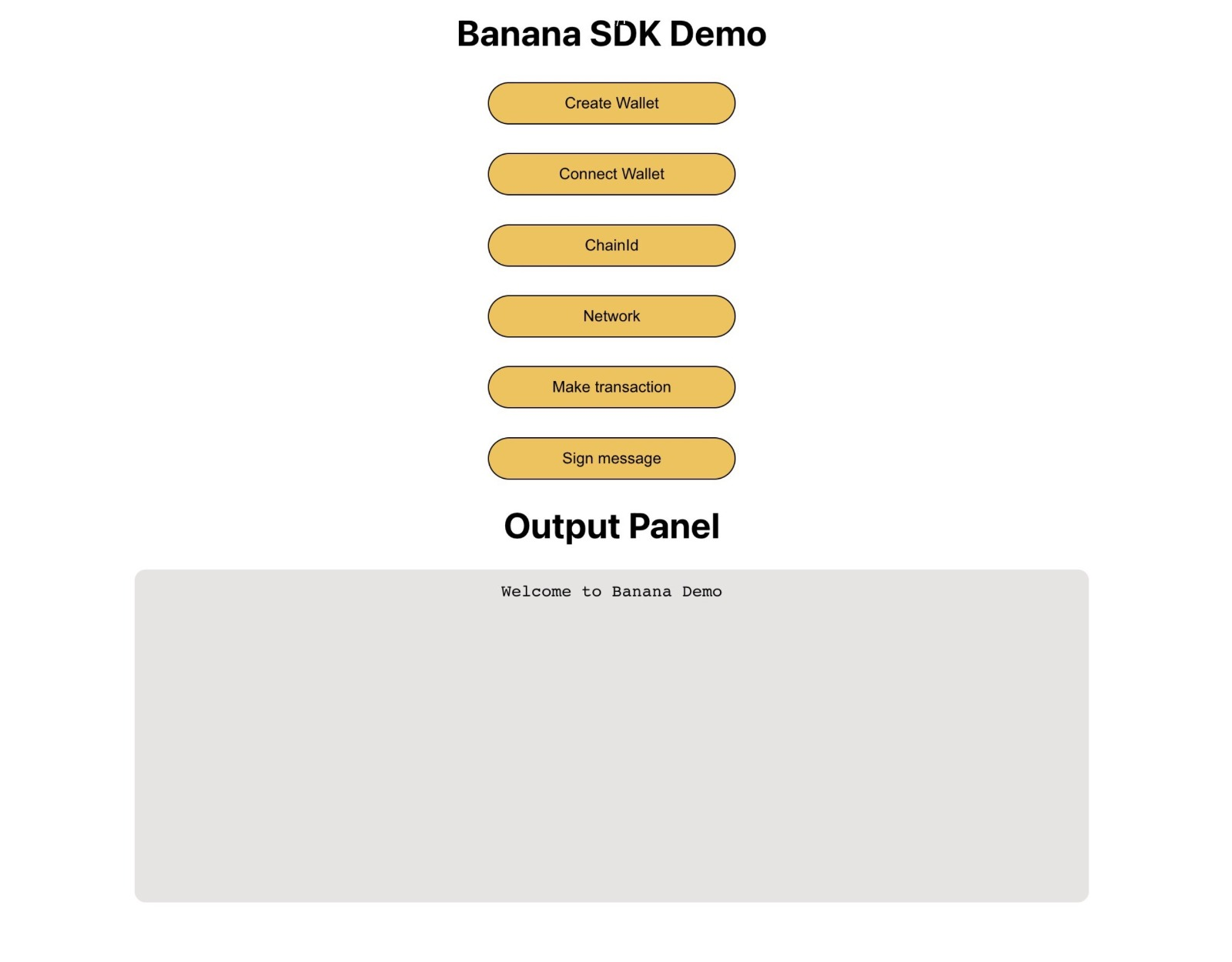
+
+For more information about building the frontend please refer to this [guide](https://banana-wallet-docs.rizelabs.io/integration/sdk-integration-tutorial/banana-less-than-greater-than-shibuya#building-the-frontend).
+
+### Step 5: Imports
+
+```
+import "./App.css";
+import { Banana, Chains } from '@rize-labs/banana-wallet-sdk';
+import { useEffect, useState } from "react";
+import { ethers } from "ethers";
+import { SampleAbi } from "./SampleAbi";
+```
+
+Download app.css and SampleAbi.js from here [App.css](https://github.com/Banana-Wallet/banana-tutorial/blob/feat/chaido-tutorial/src/App.css) and [SampleAbi.js](https://github.com/Banana-Wallet/banana-tutorial/blob/feat/chaido-tutorial/src/SampleAbi.js)
+
+Initializing some states for demo
+
+```
+const [walletAddress, setWalletAddress] = useState("");
+const [bananaSdkInstance, setBananSdkInstance] = useState(null);
+const [isLoading, setIsLoading] = useState(false);
+const [walletInstance, setWalletInstance] = useState(null);
+const [output, setOutput] = useState("Welcome to Banana Demo");
+const SampleContractAddress = "0xCB8a3Ca479aa171aE895A5D2215A9115D261A566";
+```
+
+### Step 6: Initializing Banana SDK instance and creating methods
+
+```
+// calling it in useEffect
+
+useEffect(() => {
+ getBananaInstance();
+}, []);
+
+ const getBananaInstance = () => {
+ const bananaInstance = new Banana(Chains.shibuyaTestnet);
+ setBananSdkInstance(bananaInstance);
+ };
+```
+
+For simplicity in this example we are creating an SDK instance for Shibuya testnet.
+
+Creating Wallet
+
+```
+const createWallet = async () => {
+ // starts loading
+ setIsLoading(true);
+
+ // creating wallet
+ const wallet = await bananaSdkInstance.createWallet();
+ setWalletInstance(wallet);
+
+ // getting address for wallet created
+ const address = await wallet.getAddress();
+ setWalletAddress(address);
+ setOutput("Wallet Address: " + address);
+ setIsLoading(false);
+ };
+
+```
+
+Developers need to call the `createWallet` method which will inherently ask the user for a wallet name. Once username is provided, the wallet is initialized for the user, and the method returns an instance of the wallet.
+
+Connecting wallet
+
+```
+const connectWallet = async () => {
+
+ // checking does wallet name is cached in cookie
+ const walletName = bananaSdkInstance.getWalletName();
+
+ // if cached we will use it
+ if (walletName) {
+ setIsLoading(true);
+
+ // connect wallet with cached wallet name
+ const wallet = await bananaSdkInstance.connectWallet(walletName);
+ setWalletInstance(wallet);
+
+ // extracting wallet address for display purpose
+ const address = await wallet.getAddress();
+ setWalletAddress(address);
+ setOutput("Wallet Address: " + address);
+ setIsLoading(false);
+ } else {
+ setIsLoading(false);
+ alert("You don't have wallet created!");
+ }
+ };
+
+```
+When the user wallet is created the wallet's public data is cached in the user's cookie. Once the `getWalletName` function fetches `walletName` from the cookie, we pass `walletName` into `connectWallet` which initializes and configures some wallet parameters internally, and returns a wallet instance.
+
+Get ChainId
+
+```
+ const getChainId = async () => {
+ setIsLoading(true);
+ const signer = walletInstance.getSigner();
+ const chainid = await signer.getChainId();
+ setOutput(JSON.stringify(chainid));
+ setIsLoading(false);
+ };
+```
+Getting `chainId` is pretty straight forward. Developers should extract the *signer* from the wallet and use `getChainId` to obtain the `chainId` of the current network.
+
+Get Network
+
+```
+ const getNetwork = async () => {
+ setIsLoading(true);
+ const provider = walletInstance.getProvider();
+ const network = await provider.getNetwork();
+ setOutput(JSON.stringify(network));
+ setIsLoading(false);
+ };
+```
+
+Extracting the network is as easy as it looks. Developers should extract the *provider* from the wallet and use the `getNetwork` method to obtain the chain info.
+
+Make transaction
+
+```
+ const makeTransaction = async () => {
+ setIsLoading(true);
+
+ // getting signer
+ const signer = walletInstance.getSigner();
+ const amount = "0.00001";
+ const tx = {
+ gasLimit: "0x55555",
+ to: SampleContractAddress,
+ value: ethers.utils.parseEther(amount),
+ data: new ethers.utils.Interface(SampleAbi).encodeFunctionData(
+ "stake",
+ []
+ ),
+ };
+
+ try {
+ // sending txn object via signer
+ const txn = signer.sendTransaction(tx);
+ setOutput(JSON.stringify(txn));
+ } catch (err) {
+ console.log(err);
+ }
+ setIsLoading(false);
+ };
+```
+
+To initiate a transaction you will create a transaction object. Extract *signer* from the wallet instance and initiate a transaction by passing the *transaction object* to the *send transaction* method.
+PS: Make sure your wallet is funded before you initiate transactions.
+
+Signing message
+
+```
+ const signMessage = async () => {
+ setIsLoading(true);
+ const sampleMsg = "Hello World";
+ const signer = walletInstance.getSigner();
+ const signMessageResponse = await signer.signBananaMessage(sampleMsg);
+ setOutput(JSON.stringify(signMessageResponse));
+ setIsLoading(false);
+ };
+```
+
+Signing a message is as simple as it looks. Pass a message that needs to be signed, and the method will return an object \{ messageSigned: "", signature: "" \}
+
+messageSigned: message that was signed.
+
+signature: signature for the signed message.
+
+### Step 7: Building the frontend
+
+JSX code for frontend
+
+```
+
+
Banana SDK Demo
+ {walletAddress &&
Wallet Address: {walletAddress}
}
+
+
+
+
+
+
+
Output Panel
+
+
{isLoading ? "Loading.." : output}
+
+
+```
+
+## Troubleshooting
+
+If you are facing a webpack 5 polyfill issue please try using `react-app-rewired`.
+
+```
+npm install react-app-rewired
+
+npm install stream-browserify constants-browserify crypto-browserify os-browserify path-browserify process stream-browserify buffer ethers@^5.7.2
+```
+
+create a file name `config-overrides.js` using the content below.
+```
+const { ProvidePlugin }= require("webpack")
+
+module.exports = {
+ webpack: function (config, env) {
+ config.module.rules = config.module.rules.map(rule => {
+ if (rule.oneOf instanceof Array) {
+ rule.oneOf[rule.oneOf.length - 1].exclude = [/\.(js|mjs|jsx|cjs|ts|tsx)$/, /\.html$/, /\.json$/];
+ }
+ return rule;
+ });
+ config.resolve.fallback = {
+ ...config.resolve.fallback,
+ stream: require.resolve("stream-browserify"),
+ buffer: require.resolve("buffer"),
+ crypto: require.resolve("crypto-browserify"),
+ process: require.resolve("process"),
+ os: require.resolve("os-browserify"),
+ path: require.resolve("path-browserify"),
+ constants: require.resolve("constants-browserify"),
+ fs: false
+ }
+ config.resolve.extensions = [...config.resolve.extensions, ".ts", ".js"]
+ config.ignoreWarnings = [/Failed to parse source map/];
+ config.plugins = [
+ ...config.plugins,
+ new ProvidePlugin({
+ Buffer: ["buffer", "Buffer"],
+ }),
+ new ProvidePlugin({
+ process: ["process"]
+ }),
+ ]
+ return config;
+ },
+}
+```
+Change package.json to start using `react-app-rewired` instead of `react-scripts`.
+
+```
+react-scripts start -> react-app-rewired start
+react-scripts build -> react-app-rewired build
+react-scripts test -> react-app-rewired test
+```
+
+If you are still unable to resolve the issue please post your query to Banana Discord [here](https://discord.gg/3fJajWBT3N)
+
+
+## Learn more
+
+To learn more about Banana Wallet head over to [banana docs](https://banana-wallet-docs.rizelabs.io/)
+
+Full tutorial code is available [here](https://github.com/Banana-Wallet/banana-tutorial/tree/feat/shibuya-tutorial)
+
+If your dApp already uses Rainbowkit then you can use Banana Wallet directly on Shibuya testnet. Please refer [here](https://docs.bananahq.io/integration/wallet-connectors/using-rainbowkit) for more information.
diff --git a/docs/build/build-on-layer-1/integrations/account-abstraction/biconomy/_category_.json b/docs/build/build-on-layer-1/integrations/account-abstraction/biconomy/_category_.json
new file mode 100644
index 0000000..7cd3826
--- /dev/null
+++ b/docs/build/build-on-layer-1/integrations/account-abstraction/biconomy/_category_.json
@@ -0,0 +1,4 @@
+{
+ "label": "Biconomy Account Abstraction SDK",
+ "position": 2
+}
\ No newline at end of file
diff --git a/docs/build/build-on-layer-1/integrations/account-abstraction/biconomy/biconomy-sdk.md b/docs/build/build-on-layer-1/integrations/account-abstraction/biconomy/biconomy-sdk.md
new file mode 100644
index 0000000..9adc0ad
--- /dev/null
+++ b/docs/build/build-on-layer-1/integrations/account-abstraction/biconomy/biconomy-sdk.md
@@ -0,0 +1,268 @@
+---
+sidebar_label: Quickstart
+sidebar_position: 1
+---
+
+# Quickstart: Smart Account Native Transfer
+
+In this guide, we will walk through creating a basic Node.js script using **TypeScript** with an implementation of the **Smart Account Package** from the Biconomy SDK. You will learn how to create a smart account and perform user operations by sending a native transfer of tokens.
+
+:::info
+Please note that this tutorial assumes you have Node JS installed on your computer and have some working knowledge of Node.
+:::
+
+## Environment set up
+
+We will clone a preconfigured Node.js project with TypeScript support to get started. Follow these steps to clone the repository to your local machine using your preferred command line interface:
+
+1. Open your command line interface, Terminal, Command Prompt, or PowerShell.
+2. Navigate to the desired directory where you would like to clone the repository.
+3. Execute the following command to clone the repository from the provided [GitHub link](https://github.com/bcnmy/quickstart)
+
+```bash
+git clone git@github.com:bcnmy/quickstart.git
+```
+
+Note that this is the ssh example, use http or GithubCli options if you prefer.
+
+```bash
+git clone https://github.com/bcnmy/quickstart.git
+```
+
+Once you have the repository on your local machine - start by installing all dependencies using your preferred package manager. In this tutorial we will use yarn.
+
+```bash
+yarn install
+yarn dev
+```
+After running these two commands you should see the printed statement “Hello World!” in your terminal. Any changes made to the `index.ts` file in the src directory should now automatically run in your terminal upon save.
+
+All packages you need for this guide are all configured and installed for you, check out the `package.json` file if you want to explore the dependencies.
+
+
+ Click to learn more about the packages
+
+- The account package will help you with creating smart contract accounts and an interface with them to create transactions.
+- The bundler package helps you with interacting with our bundler or alternatively another bundler of your choice.
+- The paymaster package works similarly to the bundler package in that you can use our paymaster or any other one of your choice.
+- The core types package will give us Enums for the proper ChainId we may want to use.
+- The modules package gives us access to the different modules we publish for the biconomy sdk.
+- The common package is needed by our accounts package as another dependency.
+- Finally the ethers package at version 5.7.2 will help us with giving our accounts an owner which will be our own EOA.
+
+
+
+Let’s first set up a .env file in the root of our project, this will need a Private Key of any Externally Owned Account (EOA) you would like to serve as the owner of the smart account we create. This is a private key you can get from wallets like MetaMask, TrustWallet, Coinbase Wallet etc. All of these wallets will have tutorials on how to export the Private key.
+
+```bash
+PRIVATE_KEY = "enter some private key"
+```
+
+Let’s give our script the ability to access this environment variable. Delete the console log inside of `src/index.ts` and replace it with the code below. All of our work for the remainder of the tutorial will be in this file.
+
+```typescript
+import { config } from "dotenv"
+
+config()
+```
+Now our code is configured to access the environment variable as needed.
+
+## Initialization
+
+Let’s import our bundler package, and providers from the ethers package:
+
+```typescript
+import { IBundler, Bundler } from '@biconomy/bundler'
+import { DEFAULT_ENTRYPOINT_ADDRESS } from "@biconomy/account"
+import { ethers } from 'ethers'
+import { ChainId } from "@biconomy/core-types"
+```
+
+IBundler is the typing for the Bundler class that we will create a new instance of.
+
+
+### **Initial Configuration**
+
+```typescript
+const bundler: IBundler = new Bundler({
+ bundlerUrl: 'https://bundler.biconomy.io/api/v2/80001/nJPK7B3ru.dd7f7861-190d-41bd-af80-6877f74b8f44',
+ chainId: ChainId.POLYGON_MUMBAI,
+ entryPointAddress: DEFAULT_ENTRYPOINT_ADDRESS,
+ })
+```
+
+- Now we create an instance of our bundler with the following:
+ - a bundler url which you can retrieve from the Biconomy Dashboard
+ - chain ID, in this case we’re using Polygon Mumbai testnet
+ - and default entry point address imported from the account package
+
+
+```typescript
+import { BiconomySmartAccountV2, DEFAULT_ENTRYPOINT_ADDRESS } from "@biconomy/account"
+```
+
+Update your import from the account package to also include BiconomySmartAccountV2 which is the class we will be using to create an instance of our smart account.
+
+```typescript
+const provider = new ethers.providers.JsonRpcProvider("https://rpc.ankr.com/polygon_mumbai")
+const wallet = new ethers.Wallet(process.env.PRIVATE_KEY || "", provider);
+```
+
+- We create a provider using a public RPC provider endpoint from Astar Foundation, feel free to use any service you wish.
+- Next we create an instance of the wallet associated to our Private key.
+
+One more thing we need to include before we move on is the module for our Smart account. You can learn more about modules here. In this instance we will create this smart account using the ECDSA module.
+
+First we import our Module:
+
+```typescript
+import { ECDSAOwnershipValidationModule, DEFAULT_ECDSA_OWNERSHIP_MODULE } from "@biconomy/modules";
+```
+Now let's initialize the module and pass it to our account creation config:
+
+```typescript
+async function createAccount() {
+
+ const module = await ECDSAOwnershipValidationModule.create({
+ signer: wallet,
+ moduleAddress: DEFAULT_ECDSA_OWNERSHIP_MODULE
+ })
+
+ let biconomySmartAccount = await BiconomySmartAccountV2.create({
+ signer: wallet,
+ chainId: ChainId.POLYGON_MUMBAI,
+ bundler: bundler,
+ entryPointAddress: DEFAULT_ENTRYPOINT_ADDRESS,
+ defaultValidationModule: module,
+ activeValidationModule: module
+})
+ console.log("address: ", await biconomySmartAccount.getAccountAddress())
+ return biconomySmartAccount;
+}
+
+```
+
+We create a new instance of the account using the BiconomySmartAccount class and passing it the configuration.
+
+We then await the initialization of the account and log out two values to out terminal: the owner of the account and the smart account address. The owner should be the EOA that you got your private key from and the smart account address will be a new address referring to the address of this wallet.
+:::info
+Smart accounts are counterfactual in nature. We know their address before they are even deployed. In this instance we won’t immediately deploy it, it will automatically be deployed on the first transaction it initiates and the gas needed for deployment will be added to that first transaction.
+:::
+
+:::caution
+Before continuing, now that we have our smart account address we need to fund it. Since we are using the Polygon Mumbai network head over to the Polygon Faucet and paste in your smart account address and get some test tokens! If you skip this step you might run into the [AA21 didn't pay prefund error](https://docs.biconomy.io/troubleshooting/commonerrors)!
+:::
+Once you have tokens available it is time to start constructing our first userOps for a native transfer.
+
+
+## Execute your first userOp
+
+```typescript
+async function createTransaction() {
+ console.log("creating account")
+
+ const smartAccount = await createAccount();
+
+ const transaction = {
+ to: '0x322Af0da66D00be980C7aa006377FCaaEee3BDFD',
+ data: '0x',
+ value: ethers.utils.parseEther('0.1'),
+ }
+
+ const userOp = await smartAccount.buildUserOp([transaction])
+ userOp.paymasterAndData = "0x"
+
+ const userOpResponse = await smartAccount.sendUserOp(userOp)
+
+ const transactionDetail = await userOpResponse.wait()
+
+ console.log("transaction detail below")
+ console.log(transactionDetail)
+}
+
+createTransaction()
+```
+
+Let’s move the call to create account into the create transaction function and have it assigned to the value smartAccount.
+
+Now we need to construct our transaction which will take the following values:
+
+- `to`: the address this interaction is directed towards, (in this case here is an address I own, feel free to change this to your own or send me more test tokens)
+- `data`: we are defaulting to ‘0x’ as we do not need to send any specific data for this transaction to work since it is a native transfer
+- `value`: we indicate the amount we would like to transfer here and use the `parseEther` utility function to make sure our value is formatted the way we need it to be
+
+Next up is building the userOp, feel free to add an additional log here if you would like to see how the partial userOp looks in this case. We’re also going to add “0x” for the paymasterAndData value as we just want this to be a regular transaction where the gas is paid for by the end user.
+
+Finally we send the userOp and save the value to a variable named userOpResponse and get the transactionDetail after calling `userOpResponse.wait()`. This function can optionally take a number to specify the amount of network confirmations you would like before returning a value. For example, if you passed `userOpResponse.wait(5)` this would wait for 5 confirmations on the network before giving you the necessary value.
+
+Check out the long transaction details available now in your console! You just created and executed your first userOps using the Biconomy SDK!
+
+
+ Click to view final code
+
+```typescript
+import { config } from "dotenv"
+import { IBundler, Bundler } from '@biconomy/bundler'
+import { ChainId } from "@biconomy/core-types"
+import { BiconomySmartAccountV2, DEFAULT_ENTRYPOINT_ADDRESS } from "@biconomy/account"
+import { ECDSAOwnershipValidationModule, DEFAULT_ECDSA_OWNERSHIP_MODULE } from "@biconomy/modules";
+import { ethers } from 'ethers';
+
+config()
+
+const provider = new ethers.providers.JsonRpcProvider("https://rpc.ankr.com/polygon_mumbai")
+const wallet = new ethers.Wallet(process.env.PRIVATE_KEY || "", provider);
+
+const bundler: IBundler = new Bundler({
+ bundlerUrl: 'https://bundler.biconomy.io/api/v2/80001/nJPK7B3ru.dd7f7861-190d-41bd-af80-6877f74b8f44',
+ chainId: ChainId.POLYGON_MUMBAI,
+ entryPointAddress: DEFAULT_ENTRYPOINT_ADDRESS,
+})
+
+const module = await ECDSAOwnershipValidationModule.create({
+ signer: wallet,
+ moduleAddress: DEFAULT_ECDSA_OWNERSHIP_MODULE
+})
+
+ async function createAccount() {
+ let biconomyAccount = await BiconomySmartAccountV2.create({
+ signer: wallet,
+ chainId: ChainId.POLYGON_MUMBAI,
+ bundler: bundler,
+ entryPointAddress: DEFAULT_ENTRYPOINT_ADDRESS,
+ defaultValidationModule: module,
+ activeValidationModule: module
+ })
+ console.log("address", biconomyAccount.accountAddress)
+ return biconomyAccount
+ }
+
+ async function createTransaction() {
+ const smartAccount = await createAccount();
+ try {
+ const transaction = {
+ to: '0x322Af0da66D00be980C7aa006377FCaaEee3BDFD',
+ data: '0x',
+ value: ethers.utils.parseEther('0.1'),
+ }
+
+ const userOp = await smartAccount.buildUserOp([transaction])
+ userOp.paymasterAndData = "0x"
+
+ const userOpResponse = await smartAccount.sendUserOp(userOp)
+
+ const transactionDetail = await userOpResponse.wait()
+
+ console.log("transaction detail below")
+ console.log(`https://mumbai.polygonscan.com/tx/${transactionDetail.receipt.transactionHash}`)
+ } catch (error) {
+ console.log(error)
+ }
+ }
+
+ createTransaction()
+```
+
+
+
+Now that you have completed our quickstart take a look at exploring further usecases in our Quick Explore guide or our Node JS guides!
diff --git a/docs/build/build-on-layer-1/integrations/account-abstraction/biconomy/images/contracts/add_contract.png b/docs/build/build-on-layer-1/integrations/account-abstraction/biconomy/images/contracts/add_contract.png
new file mode 100644
index 0000000..adb1f34
Binary files /dev/null and b/docs/build/build-on-layer-1/integrations/account-abstraction/biconomy/images/contracts/add_contract.png differ
diff --git a/docs/build/build-on-layer-1/integrations/account-abstraction/biconomy/images/contracts/changes.png b/docs/build/build-on-layer-1/integrations/account-abstraction/biconomy/images/contracts/changes.png
new file mode 100644
index 0000000..ec09718
Binary files /dev/null and b/docs/build/build-on-layer-1/integrations/account-abstraction/biconomy/images/contracts/changes.png differ
diff --git a/docs/build/build-on-layer-1/integrations/account-abstraction/biconomy/images/contracts/new_contract.png b/docs/build/build-on-layer-1/integrations/account-abstraction/biconomy/images/contracts/new_contract.png
new file mode 100644
index 0000000..3cb663a
Binary files /dev/null and b/docs/build/build-on-layer-1/integrations/account-abstraction/biconomy/images/contracts/new_contract.png differ
diff --git a/docs/build/build-on-layer-1/integrations/account-abstraction/biconomy/images/fiat-1.png b/docs/build/build-on-layer-1/integrations/account-abstraction/biconomy/images/fiat-1.png
new file mode 100644
index 0000000..1e291c4
Binary files /dev/null and b/docs/build/build-on-layer-1/integrations/account-abstraction/biconomy/images/fiat-1.png differ
diff --git a/docs/build/build-on-layer-1/integrations/account-abstraction/biconomy/images/keys/paymaster_keys.png b/docs/build/build-on-layer-1/integrations/account-abstraction/biconomy/images/keys/paymaster_keys.png
new file mode 100644
index 0000000..8f85fb6
Binary files /dev/null and b/docs/build/build-on-layer-1/integrations/account-abstraction/biconomy/images/keys/paymaster_keys.png differ
diff --git a/docs/build/build-on-layer-1/integrations/account-abstraction/biconomy/images/overview/censored.jpeg b/docs/build/build-on-layer-1/integrations/account-abstraction/biconomy/images/overview/censored.jpeg
new file mode 100644
index 0000000..0e15a5c
Binary files /dev/null and b/docs/build/build-on-layer-1/integrations/account-abstraction/biconomy/images/overview/censored.jpeg differ
diff --git a/docs/build/build-on-layer-1/integrations/account-abstraction/biconomy/images/overview/erc20gas.png b/docs/build/build-on-layer-1/integrations/account-abstraction/biconomy/images/overview/erc20gas.png
new file mode 100644
index 0000000..709cb8b
Binary files /dev/null and b/docs/build/build-on-layer-1/integrations/account-abstraction/biconomy/images/overview/erc20gas.png differ
diff --git a/docs/build/build-on-layer-1/integrations/account-abstraction/biconomy/images/overview/fullstackaa.png b/docs/build/build-on-layer-1/integrations/account-abstraction/biconomy/images/overview/fullstackaa.png
new file mode 100644
index 0000000..57c21b0
Binary files /dev/null and b/docs/build/build-on-layer-1/integrations/account-abstraction/biconomy/images/overview/fullstackaa.png differ
diff --git a/docs/build/build-on-layer-1/integrations/account-abstraction/biconomy/images/overview/fulstackaa.png b/docs/build/build-on-layer-1/integrations/account-abstraction/biconomy/images/overview/fulstackaa.png
new file mode 100644
index 0000000..4255b43
Binary files /dev/null and b/docs/build/build-on-layer-1/integrations/account-abstraction/biconomy/images/overview/fulstackaa.png differ
diff --git a/docs/build/build-on-layer-1/integrations/account-abstraction/biconomy/images/overview/modularsa.png b/docs/build/build-on-layer-1/integrations/account-abstraction/biconomy/images/overview/modularsa.png
new file mode 100644
index 0000000..c8bd009
Binary files /dev/null and b/docs/build/build-on-layer-1/integrations/account-abstraction/biconomy/images/overview/modularsa.png differ
diff --git a/docs/build/build-on-layer-1/integrations/account-abstraction/biconomy/images/overview/overview.webp b/docs/build/build-on-layer-1/integrations/account-abstraction/biconomy/images/overview/overview.webp
new file mode 100644
index 0000000..1656158
Binary files /dev/null and b/docs/build/build-on-layer-1/integrations/account-abstraction/biconomy/images/overview/overview.webp differ
diff --git a/docs/build/build-on-layer-1/integrations/account-abstraction/biconomy/images/overview/sponsored.png b/docs/build/build-on-layer-1/integrations/account-abstraction/biconomy/images/overview/sponsored.png
new file mode 100644
index 0000000..043f649
Binary files /dev/null and b/docs/build/build-on-layer-1/integrations/account-abstraction/biconomy/images/overview/sponsored.png differ
diff --git a/docs/build/build-on-layer-1/integrations/account-abstraction/biconomy/images/paymaster/add_paymaster.png b/docs/build/build-on-layer-1/integrations/account-abstraction/biconomy/images/paymaster/add_paymaster.png
new file mode 100644
index 0000000..72c98d7
Binary files /dev/null and b/docs/build/build-on-layer-1/integrations/account-abstraction/biconomy/images/paymaster/add_paymaster.png differ
diff --git a/docs/build/build-on-layer-1/integrations/account-abstraction/biconomy/images/paymaster/dashboard_login.png b/docs/build/build-on-layer-1/integrations/account-abstraction/biconomy/images/paymaster/dashboard_login.png
new file mode 100644
index 0000000..900dcdf
Binary files /dev/null and b/docs/build/build-on-layer-1/integrations/account-abstraction/biconomy/images/paymaster/dashboard_login.png differ
diff --git a/docs/build/build-on-layer-1/integrations/account-abstraction/biconomy/images/paymaster/deposit_funds.png b/docs/build/build-on-layer-1/integrations/account-abstraction/biconomy/images/paymaster/deposit_funds.png
new file mode 100644
index 0000000..91919fb
Binary files /dev/null and b/docs/build/build-on-layer-1/integrations/account-abstraction/biconomy/images/paymaster/deposit_funds.png differ
diff --git a/docs/build/build-on-layer-1/integrations/account-abstraction/biconomy/images/paymaster/first_time_gastank.png b/docs/build/build-on-layer-1/integrations/account-abstraction/biconomy/images/paymaster/first_time_gastank.png
new file mode 100644
index 0000000..5ce42cd
Binary files /dev/null and b/docs/build/build-on-layer-1/integrations/account-abstraction/biconomy/images/paymaster/first_time_gastank.png differ
diff --git a/docs/build/build-on-layer-1/integrations/account-abstraction/biconomy/images/paymaster/register_paymaster.png b/docs/build/build-on-layer-1/integrations/account-abstraction/biconomy/images/paymaster/register_paymaster.png
new file mode 100644
index 0000000..722bb39
Binary files /dev/null and b/docs/build/build-on-layer-1/integrations/account-abstraction/biconomy/images/paymaster/register_paymaster.png differ
diff --git a/docs/build/build-on-layer-1/integrations/account-abstraction/biconomy/images/paymaster/withdraw_funds.png b/docs/build/build-on-layer-1/integrations/account-abstraction/biconomy/images/paymaster/withdraw_funds.png
new file mode 100644
index 0000000..ab34ecc
Binary files /dev/null and b/docs/build/build-on-layer-1/integrations/account-abstraction/biconomy/images/paymaster/withdraw_funds.png differ
diff --git a/docs/build/build-on-layer-1/integrations/account-abstraction/biconomy/index.md b/docs/build/build-on-layer-1/integrations/account-abstraction/biconomy/index.md
new file mode 100644
index 0000000..78b336a
--- /dev/null
+++ b/docs/build/build-on-layer-1/integrations/account-abstraction/biconomy/index.md
@@ -0,0 +1,43 @@
+import Figure from '/src/components/figure'
+
+# Overview
+
+The Biconomy SDK is an Account Abstraction toolkit that enables the simplest UX on your dApp, wallet or appchain. Built on top of the ERC 4337 solution for Account Abstraction, we offer a full-stack solution for tapping into the power of our Smart Accounts Platform, Paymasters, and Bundlers.
+
+
+
+## Smart Accounts Platform
+
+The Biconomy Smart Account is an ERC 4337-compliant solution that works with any Paymaster and Bundler service. Smart Accounts are governed by code instead of ECDSA, which allows for other types of signature algorithms to be used with the Smart Account. Additionally, the smart accounts package allows you to quickly and easily build and execute User Operations or userOps. These are pseudo-transaction objects that eventually execute as a transaction on the EVM.
+
+The Biconomy Smart Accounts are signer agnostic, which allows you to use any authorization package of your choice as long as you can pass a signer to our SDK upon the creation of a Smart Account. Check out different ways you can create a Biconomy Smart Account [here](https://docs.biconomy.io/category/signers).
+
+
+Smart Accounts are further enhanced by validation modules that allow you to execute arbitrary logic before validating a userOp. This allows you, as a developer, to build modules that allow for session keys, multi-chain validation modules, pass keys, and more.
+
+
+
+If you want to start diving into Smart Accounts you can do so [here](https://docs.biconomy.io/category/smart-accounts). If you know all about Smart Accounts and prefer to start working with modules, start learning about them [here](https://docs.biconomy.io/category/modules) or follow this step-by-step [tutorial](https://docs.biconomy.io/category/session-keys-tutorial) on how to build a dApp that utilizes session key modules.
+
+## Paymaster
+
+Biconomy offers a Paymaster service designed with one of the best developer experiences in mind. Simply use one URL and switch modes between our sponsorship paymaster and our Token Paymaster.
+
+### Sponsorship Paymaster
+
+
+
+
+If the mode you choose in the request to the Paymaster URL is the sponsored mode, your users will benefit from gasless transactions, and you remove the friction point of needed native tokens to pay for gas on transactions. Learn how to set up your paymaster [here](https://docs.biconomy.io/dashboard/paymaster).
+
+### Token Paymaster
+
+
+
+Switching the mode of your Paymster to ERC20 allows you to unlock an experience where users can pay gas in any of our supported ERC20 tokens on different networks. Check out the latest supported tokens [here](https://docs.biconomy.io/supportedchains/supportedTokens).
+
+Learn how to utilize either of these Paymasters by checking out our How To Guide on [Executing transactions](https://docs.biconomy.io/category/executing-transactions)
+
+## Bundler
+
+The Bundler is a service that tracks userOps that exist in an alternative mem pool and as the name suggests, bundles them together to send to an Entry Point Contract for eventual execution onchain. This is the final piece of the flow where after constructing your userOp and then potentially signing it with data from a paymaster, you send the userOp on chain to be handled and executed as a transaction on the EVM. You can start using our Bundlers right now in your dApps; each of our [tutorials](https://docs.biconomy.io/category/tutorials) will walk you through how to use them in different scenarios.
\ No newline at end of file
diff --git a/docs/build/build-on-layer-1/integrations/account-abstraction/index.md b/docs/build/build-on-layer-1/integrations/account-abstraction/index.md
new file mode 100644
index 0000000..229dc55
--- /dev/null
+++ b/docs/build/build-on-layer-1/integrations/account-abstraction/index.md
@@ -0,0 +1,27 @@
+# Account Abstraction
+
+:::note
+Please note that this section is under active development.
+:::
+
+## Overview
+Here you will find all the information required to refine the end-user experience and allow for seamless web2-like interactions with dApps and accounts on the Astar EVM.
+
+Account Abstraction is a blockchain technology that enables users to utilize smart contracts as their accounts. While the default account for most users is an Externally Owned Account (EOA), which is controlled by an external private key, it requires users to have a considerable understanding of blockchain technology to use them securely. Fortunately, smart contract accounts can create superior user experiences.
+
+### Using Account Abstraction
+There are two primary ways users can use account abstraction: with third party meta transaction services or by sending ERC-4337 transactions.
+
+#### Meta Transactions
+Meta transactions are bespoke third party services for achieving account abstraction.
+
+#### ERC-4337
+ERC-4337, also known as EIP-4337.
+- Banana Wallet SDK
+
+
+
+import DocCardList from '@theme/DocCardList';
+import {useCurrentSidebarCategory} from '@docusaurus/theme-common';
+
+
diff --git a/docs/build/build-on-layer-1/introduction/create_account.md b/docs/build/build-on-layer-1/introduction/create_account.md
index 012388e..7415431 100644
--- a/docs/build/build-on-layer-1/introduction/create_account.md
+++ b/docs/build/build-on-layer-1/introduction/create_account.md
@@ -3,8 +3,8 @@ sidebar_position: 3
---
# Create Account
-If you never created a native Astar account, please follow the instructions in the [User Guide] (/docs/use/how-to-guides/layer-1/manage-wallets/create-wallet.md) [INSERT LINK].
+If you've never created a native Astar account, please follow the instructions in the [User Guide] (/docs/use/how-to-guides/layer-1/manage-wallets/create-wallet.md) [INSERT LINK].
-If you are building EVM smart contracts you will need Metamask. Watch this short video to learn how.
+If you are building EVM smart contracts you will need MetaMask. Watch this short video to learn how.
diff --git a/docs/build/build-on-layer-1/smart-contracts/building-cross-chain-contracts/xcm/using-xcm/_category_.json b/docs/build/build-on-layer-1/smart-contracts/building-cross-chain-contracts/xcm/using-xcm/_category_.json
deleted file mode 100644
index 53d6cac..0000000
--- a/docs/build/build-on-layer-1/smart-contracts/building-cross-chain-contracts/xcm/using-xcm/_category_.json
+++ /dev/null
@@ -1,4 +0,0 @@
-{
- "label": "Using XCM",
- "position": 1
-}
\ No newline at end of file
diff --git a/docs/build/build-on-layer-1/smart-contracts/building-cross-chain-contracts/xcm/using-xcm/index.md b/docs/build/build-on-layer-1/smart-contracts/building-cross-chain-contracts/xcm/using-xcm/index.md
deleted file mode 100644
index 7864bf9..0000000
--- a/docs/build/build-on-layer-1/smart-contracts/building-cross-chain-contracts/xcm/using-xcm/index.md
+++ /dev/null
@@ -1,18 +0,0 @@
-# XCM
-
-Astar Network stands as a flexible and highly interoperable decentralized application hub supporting not only WebAssembly and EVM smart contracts, but cross-VM communications (XVM), as well.
-Astar commonly utilize XCM to leverage assets from other parachains. Furthermore, XCM finds utility within smart contracts to build purely cross-chain dApps, for deployment on either EVM or Wasm stack, or both.
-
-
-
-The most frequently encountered scenario involves users transferring their **DOT** from Polkadot over to **Astar**, and vice-versa. The Astar ecosystem version of **DOT** can then be used for smart contracts, and dApps can integrate it. Another commonly observed application is within DeFi dApps, where XCM enables **DOT** trading without requiring a bridge, and offer asset swaps across multiple chains that would have previously required many steps, requiring only one transaction.
-
-In this section, we will describe the technical details of XCM so that developers can leverage it in their dApps. Do note that Astar/Polkadot and Shiden/Kusama examples are interchangeable as the features are supported on both networks.
-
-
-
-import DocCardList from '@theme/DocCardList';
-import {useCurrentSidebarCategory} from '@docusaurus/theme-common';
-
-
-